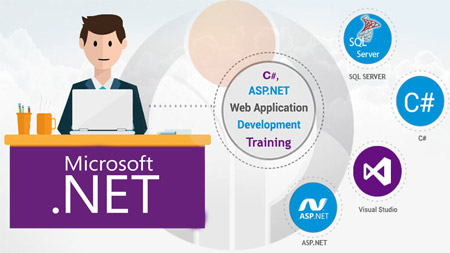
Learning Outcome
Course Covers
Learning Outcome
- Describe the core syntax and features of C#.
- Create and call methods, catch and handle exceptions, and describe the monitoring requirements of large-scale applications.
- Implement the basic structure and essential elements of a typical desktop application.
- Create classes, define and implement interfaces, and create and use generic collections.
- Use inheritance to create a class hierarchy, extend a .NET Framework class, and create generic classes and methods.
- Read and write data by using file input/output and streams, and serialize and deserialize data in different formats.
- Read and write data by using ADO.Net.
Course Covers
.NET Framework Overview
- .NET Platform architecture
- .NET Framework architecture overview
- Common Language Runtime (CLR)
- .Net Assemblies and execution model
- Common Type System (CTS)
- Framework Class Library (FCL)
.NET Framework Architecture
- .NET Platform architecture
- .NET Framework architecture
- Common language Runtime (CLR)
- Intermediate Language (IL)
- .NET execution model
- Common Language Specification (CLS)
- Common Type System (CTS)
- Language Integration
- Visual Studio .NET development environment
Introduction to C# language
- Primitive data types
- Enumerations
- Expressions
- Statements
- Control structures (if, for, while, do..while, foreach)
- Methods
Object-oriented concepts in .NET
- Classes and interfaces
- Structs
- Constructors, methods, fields, constants, access modifiers
- Inheritance and polymorphism
- Properties, indexers, operators
- Static methods and constructors
- Namespaces
- Exceptions handling
Common type system (CTS)
- System.Object, equality, hash codes, cloning
- Value types & reference types, boxing and unboxing
- Type conversions and casting
- Enums and flags
Delegates and events
- Delegates and multicast delegates
- Events
Attributes
- Using attributes
- Creating custom attributes
Arrays and collections
- Arrays
- Jagged vs. multidimensional arrays
- Collections and enumerators
- Lists, dictionaries, hash tables, sorted lists
Working with strings
- Strings
- Strings manipulation (StringBuilder)
- String formatting
- Unicode, encodings, globalization and internationalization
Regular expressions
- Regular expressions language
- Searching, extracting, validating and replacing text by regular expressions
Memory and resource management
- Managed heap and garbage collection
- Destructors and finalizers
Input/output
- Streams, readers/writers
- Files and directories
- Asynchronous input/output
Data access with ADO.NET
- Connected V/s Disconned Archtiecture
- Basic concepts and classes (Connection, Command, DataReader, DataAdapter, CommandBuilder)
- Data sets, data tables, relations, views, constraints
- Strongly-typed data sets
Windows Forms
- Windows Forms programming model, basic classes (Component, Control, ScrollableControl, ContainerControl)
- Forms and dialogs
- Basic controls (Label, TextBox, Button)
- Adding controls to forms
- Handling events
- Advanced controls (menus, status bars, toolbars)
- Data binding and DataGrid
- Custom controls
Course Duration:
.NET Course Duration
Track | Regular Track | Weekend Track | Fast Track |
---|---|---|---|
Course Duration | 45 – 60 Days | 8 Weekends | 5 Days |
Hours | 2 hours a day | 3 hours a day | 6+ hours a day |
Training Mode | Live Classroom | Live Classroom | Live Classroom |
Online and Offline classes available