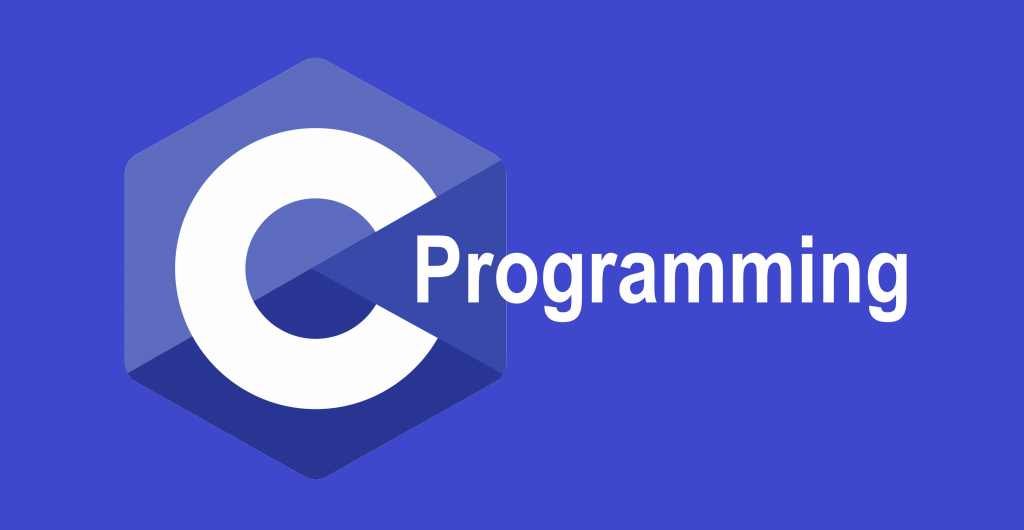
Learning Outcome
Course Covers
Learning Outcome
- Understand the concept of programming in C
- Start building your C programs
- Printing your program on screen
- Perform some mathematical or arithmetical operations.
Course Covers
1. Introduction to C
- History
- Features
- Rules for C Programming
- Writing C Program
- Introduction to Function
- Printf() & Scanf()
- Header files
- Pre-processor directives
- Creating a file
- Saving a file
- Compiling program
- Running a program
- Editor Block Commands
- Selection
- Copy
- Delete
- Move
- De-select
- Undo
- Comments
- Data Types
- Primary
- Modified (Modifiers)
- Derived
- Variables
- Variable naming rules
- Variable Declaration
- Variable Assignment
- Format Specifiers
- Reading Data from Keyboard
- scanf
- gets
- getchar
- getch
- getche
- fflush
2. Operators
- Arithmetic Operators
- Logical Operators
- Relational Operators
- Bitwise Operators
- Unary Operators
- Ternary Operators
- Concept of true and false
- Difference between & and &&
- Difference between | and||
- Difference between = and ==
3. Conditional Statements
- Simple If Statement
- Else if Statement
- Nested If Statement
- Exercise
4. Looping Statement
- FOR Statement
- WHILE Statement
- DO_WHILE Statement
- Break and Continue
- Exercise
5. Arrays
- Array Initialization
- One Dimensional
- Two Dimensional
- Three Dimensional
- Matrix Operations
- Address Calculations
6. String Operations
- Length
- Reverse
- Palindrome
7. Pointers
- Introduction
- Pointer Types
- Pointers to Strings
- Pointers to Array
- Pointers to Structure
- Pointers and Dynamic Allocation of Memory
- Pointers to function
- Array of Pointers
- Command Line Argument
8. Functions
- In-Build Functions
- Mathematical
- String
- Character
- User Defined Functions (UDF)
- Function Declaration
- Function Definition
- Function Call
- Recursion
- Pass by Value
- Pass by Address
- Pass by Reference
9. Macros
- Macros Vs Functions
- Multiline Macros
- Line Continuation Character
10. Scope of Variables
11. Storage Classes
- Automatic
- Register
- Static
- Extern
12. Structures
- Structure within structure
- typedef keyword
- Difference between typedef and Macr
- Pointer to Structure
- Arrow Operator ->
13. File Handling
- Text Mode
- Binary Mode
C Language Course Duration
Track | Regular Track | Weekend Track | Fast Track |
---|---|---|---|
Course Duration | 45 – 60 Days | 8 Weekends | 5 Days |
Hours | 2 hours a day | 3 hours a day | 6+ hours a day |
Training Mode | Live Classroom | Live Classroom | Live Classroom |
Online and Offline Mode Available