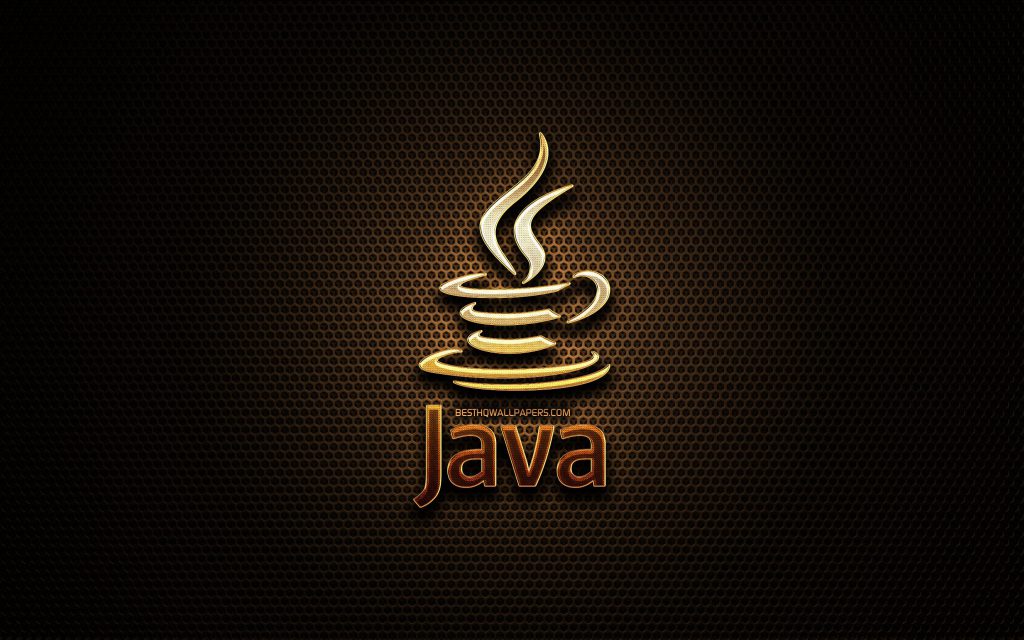
Identify core aspects of object-oriented programming and features of the Java language.
Use Eclipse for writing and running Java code.
Develop programs that use Java collections and apply core object-oriented programming concepts using classes, polymorphism, and method overloading.
Test code by applying principles of test-driven development using Java’s unit testing framework.
Unit 1: Introduction to Java programming
- The Java Virtual Machine
- Arrays
- Conditional and looping constructs
- Variables and data types
Unit 2: Object-oriented programming with Java Classes and Objects
- Fields and Methods
- Constructors
- Nested classes
- Garbage collection
- Overloading methods
Unit 3: Inheritance
- Overriding methods
- Interfaces
- Making methods and classes final
- Polymorphism
- Abstract classes and methods
Unit 4: Exception handling with try-throw-catch-finally constructs
- The Exception class
Unit 5: The Object class
- Cloning objects
- Strings
- The JDK LinkedList class
- String conversions
Unit 6: Working with types: Wrapper classes
- Enumeration interface
Unit 7: Packages
- Documentation comments
- Package access
Unit 8: Applets
- Applet capabilities and restrictions
- Configuring applets
Unit 9: Basics of AWT and Swing
- Layout Managers
- Event Handling
- Panels
- Classes for various controls, such as labels, choice, list, Checkbox, etc.
- The Action Listener interface
- Using menus
- Using the adapter classes
- Graphics
- Dialogs and frames
Unit 10: Threads
- Synchronization
Unit 11: The I/O Package
- Reader and Writer classes
- InputStream and OutputStream classes
Unit 12: Basic concepts of networking
- Concepts of URLs
- Working with URLs
- Sockets
Unit 13: Database Connectivity with JDBC
- Java Security
Java/Advance Java Course Duration
Track | Regular Track | Weekend Track | Fast Track |
---|---|---|---|
Course Duration | 45 – 60 Days | 8 Weekends | 5 Days |
Hours | 2 hours a day | 3 hours a day | 6+ hours a day |
Training Mode | Live Classroom | Live Classroom | Live Classroom |
Online and Offline Classes available